♥️24
Ich mag das.


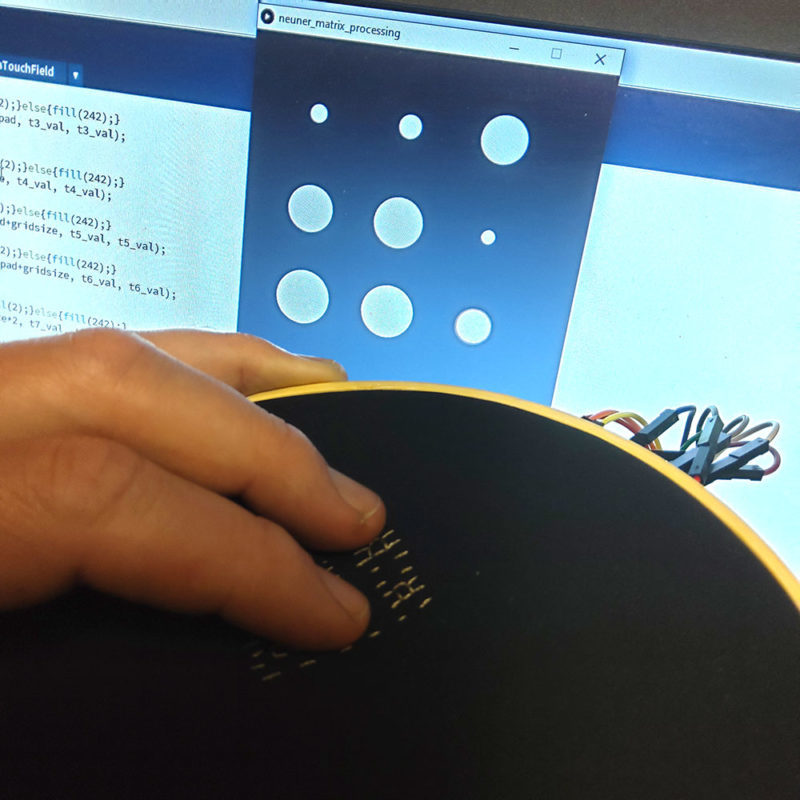
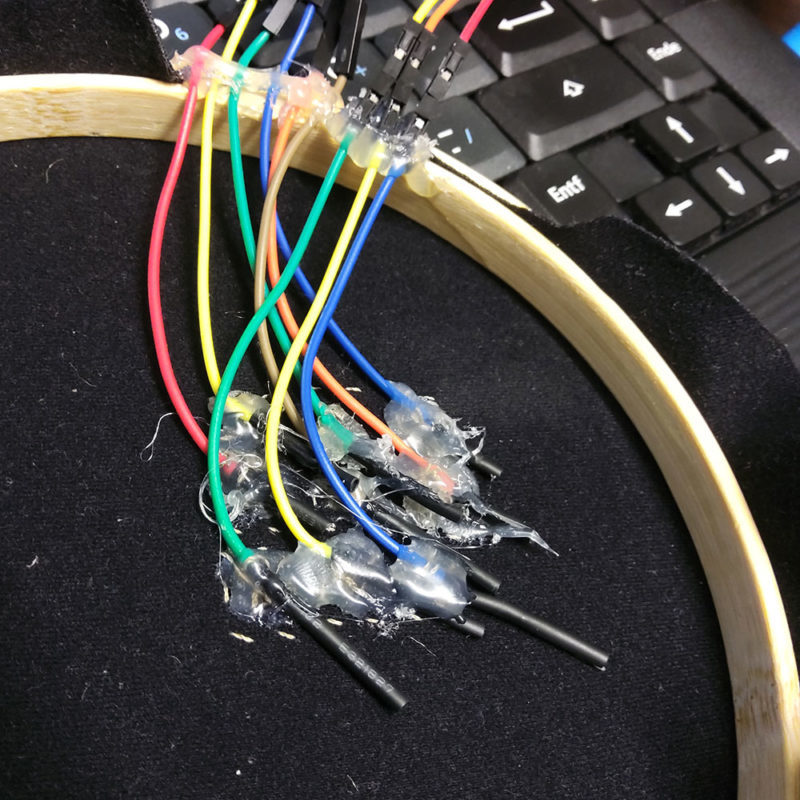
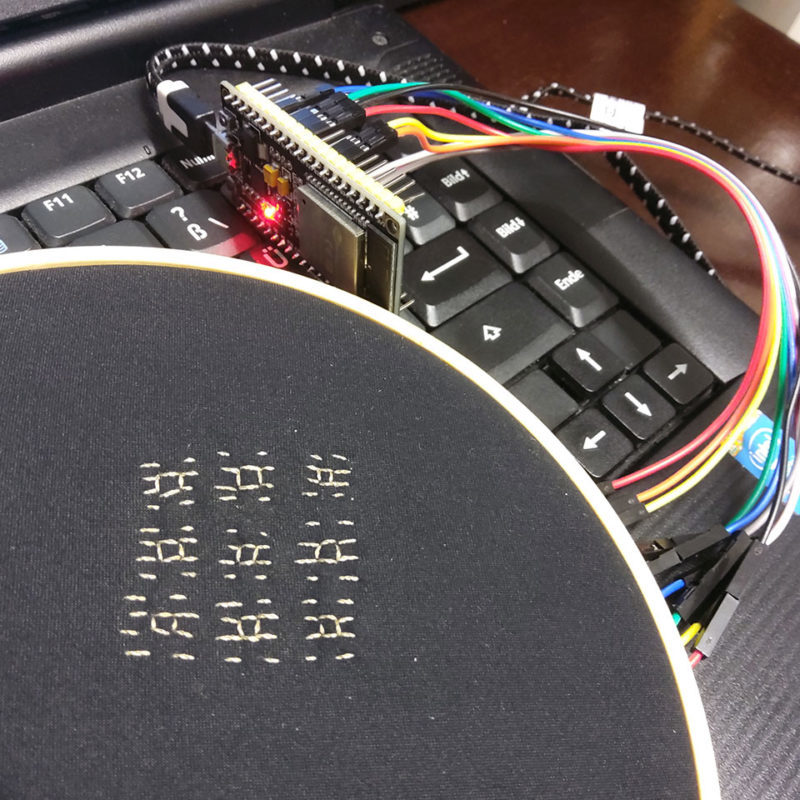
CODE FOR PROCESSING
import processing.serial.*;
int t1_val = 0;
int t2_val = 0;
int t3_val = 0;
boolean t1_triggered = false;
boolean t2_triggered = false;
boolean t3_triggered = false;
int gridsize = 10;
int pad = 80;
Serial myPort;
void setup () {
size(400,400); // window size
ellipseMode(CENTER);
// List all the available serial ports
//println(Serial.list());
String portName = Serial.list()[1];
myPort = new Serial(this, portName, 9600);
myPort.bufferUntil('\n');
gridsize = int((width-pad )*.3333);
myPort.write('s');
}
void serialEvent(Serial myport) {
String portData = myport.readString();
String[] stringData = split(portData, ',');
float[] values = float(stringData);
t1_val = int(values[0]);
t2_val = int(values[1]);
t3_val = int(values[2]);
if(int(values[3]) == 1 ){t1_triggered=true; }else{ t1_triggered = false; }
if(int(values[4]) == 1 ){t2_triggered=true; }else{ t2_triggered = false; }
if(int(values[5]) == 1 ){t3_triggered=true; }else{ t3_triggered = false; }
myPort.write('s');
}
void draw(){
noStroke();
background(222);
fill(22);
if(t1_triggered){fill(2);}else{fill(142);}
ellipse(pad,pad, t1_val, t1_val);
if(t2_triggered){fill(2);}else{fill(142);}
ellipse(pad+gridsize,pad, t2_val, t2_val);
if(t3_triggered){fill(2);}else{fill(142);}
ellipse(pad+gridsize*2,pad, t3_val, t3_val);
}
CODE FOR ARDUINO/ESP32
// ----------------------------------
// -------MAIN LOOP -----------------
// ----------------------------------
// initalize the aTouch objects : aTouch namewahtevet(yourpinnumber);
aTouch touch1(2);
aTouch touch2(4);
aTouch touch3(12);
void setup() {
Serial.begin(9600);
Serial.println("0,0,0");
}
void loop() {
// simply read input and do some calc
touch1.readAndProcessInput();
touch2.readAndProcessInput();
touch3.readAndProcessInput();
if (Serial.available()>0){
Serial.print(touch1.smoothed_val);
Serial.print(",");
Serial.print(touch2.smoothed_val);
Serial.print(",");
Serial.print(touch3.smoothed_val);
Serial.print(",");
if(touch1.is_triggered){Serial.print("1");}else{Serial.print("2");}
Serial.print(",");
if(touch2.is_triggered){Serial.print("1");}else{Serial.print("2");}
Serial.print(",");
if(touch3.is_triggered){Serial.println("1");}else{Serial.println("2");}
}
delay(30);
}
// ----------------------------------
// ------- TRXYS TOUCH HELPERS ------
// ----------------------------------
// ( put this code PRIOR to your mainloop code or you will get nice errors :) )
// simple lerp helper function
float return_lerp(float _s, int _target,int _time){
_s = _s + (( float(_target) - _s)/float(_time));
return _s;
}
class aTouch {
private:
bool prev_touch_state = false;
byte pin;
int smooth_time = 3;
int trigger_threshold = 15;
long ts = 0;
public:
int current_val = 0;
int smoothed_val = 0;
int diff_val = 0;
bool is_triggered = false;
bool on_pressed = false;
bool on_released = false;
bool is_holded = false;
aTouch(byte pin) {
this->pin = pin;
}
void readAndProcessInput() {
// reset interaction states
on_pressed = false;
on_released = false;
// directly read out values TWICE = BUGFIX for debouncing
current_val = touchRead(pin);
delayMicroseconds(10);
current_val = touchRead(pin);
//calculate smoothed input values
smoothed_val = return_lerp(smoothed_val,current_val,smooth_time);
// calc current differential sum of button
diff_val = smoothed_val - current_val;
// check if there is a noticable difference input values
if( diff_val > trigger_threshold){
if(prev_touch_state == false){
is_triggered = true;
prev_touch_state = is_triggered;
on_pressed = true;
ts = millis(); // set timestamp for hold routine
}
}else if( diff_val < trigger_threshold*-.4){
if(prev_touch_state == true){
is_triggered = false;
prev_touch_state = is_triggered;
on_released = true;
}
}
// calculate timed holding function
if( ts + 2500 < millis() && is_triggered){
is_holded = true;
}else{
is_holded = false;
}
}
void printAllMetadata() {
// only use this on one touchinput only - it will skrew up else
Serial.print (on_pressed);
Serial.print(",");
Serial.print (on_released);
Serial.print(",");
Serial.print (is_triggered);
Serial.print(",");
Serial.print (current_val);
Serial.print(",");
Serial.println(smoothed_val);
}
};